using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace STUDY.R_P_S
{
class Player_User
{
private int user_num;
public int user_num_gs
{
get
{
return user_num;
}
set
{
user_num = value;
}
}
}
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace STUDY.R_P_S
{
class Computer
{
private int com_num;
public int com_num_gs
{
get
{
Random r = new Random();
com_num = r.Next(1, 4);
return com_num;
}
set
{
com_num = value;
}
}
}
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace STUDY.R_P_S
{
class Game
{
private int input_num;
public int input_num_gs
{
get
{
return input_num;
}
set
{
input_num = value;
}
}
public void menu()
{
Console.WriteLine("·Rock Paper Scissors Game!");
while (true)
{
Console.WriteLine("·Rock [1]");
Console.WriteLine("·Paper [2] ");
Console.WriteLine("·Scissors [3]");
Console.WriteLine("·E X I T [0]");
Console.WriteLine("");
do
{
Console.Write("·Selection Number --> ");
try
{
input_num = Int32.Parse(Console.ReadLine());
if (input_num == 0)
{
Console.WriteLine("Let's end the game");
Console.WriteLine("Thank you.");
Environment.Exit(0);
}
else if (input_num > 3 || input_num < 0)
{
Console.WriteLine("--> Error! Unlisted selection!");
Console.WriteLine("");
}
}
catch (Exception e)
{
Console.WriteLine("--> Error! You have entered something other than a number!");
Console.WriteLine("");
}
} while (input_num > 3 || input_num < 0);
int user_num;
String user_str = "";
int com_num;
String com_str = "";
String result = "";
Player_User pu = new Player_User();
pu.user_num_gs = input_num;
user_num = pu.user_num_gs;
Computer com = new Computer();
com_num = com.com_num_gs;
//User
if (user_num == 1)
{
user_str = "Rock";
}
else if (user_num == 2)
{
user_str = "Paper";
}
else
{
user_str = "Scissors";
}
//Computer
if (com_num == 1)
{
com_str = "Rock";
}
else if (com_num == 2)
{
com_str = "Paper";
}
else
{
com_str = "Scissors";
}
//Result
switch (user_num - com_num)
{
case -2:
result = "WIN";
break;
case -1:
result = "LOSE";
break;
case 0:
result = "DRAW";
break;
case 1:
result = "WIN";
break;
case 2:
result = "LOSE";
break;
}
Console.WriteLine("");
Console.WriteLine("[ User : " + user_str + "] " + " VS " + " [ Computer : " + com_str + " ]");
Console.WriteLine("--> Result : " + result);
Console.WriteLine("");
}
}
}
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace STUDY.R_P_S
{
class Program
{
static void Main(string[] args)
{
Game rps = new Game();
rps.menu();
}
}
}
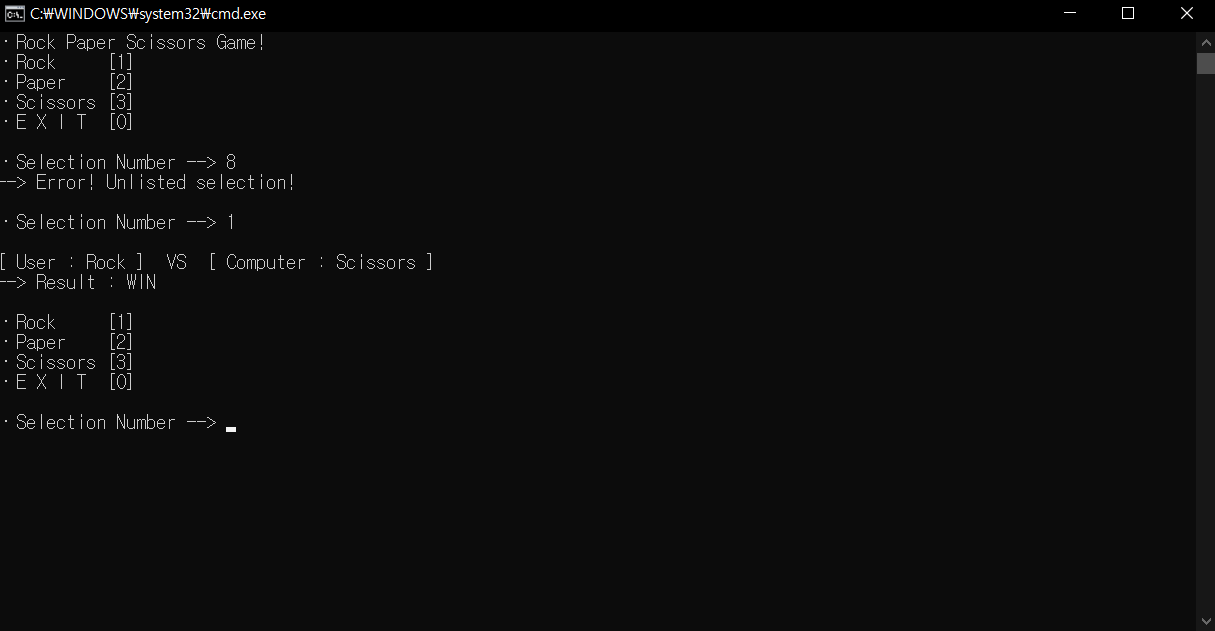
'C# 개인 공부 기록용 > 개인 실습' 카테고리의 다른 글
DataGridView + Selected (Cell, Row) (0) | 2022.07.18 |
---|---|
XML + ORACLE DB + SQL QUERY (0) | 2022.07.13 |
Oracle DB Login Form + SQL QUERY (OracleConnection + SQL QUERY) (0) | 2022.07.11 |
Oracle DB Login Form (OracleConnection) (0) | 2022.07.08 |
숫자 퀴즈 게임 (Number Quiz Game) (0) | 2022.07.03 |